dotted line drawings 3d for mom
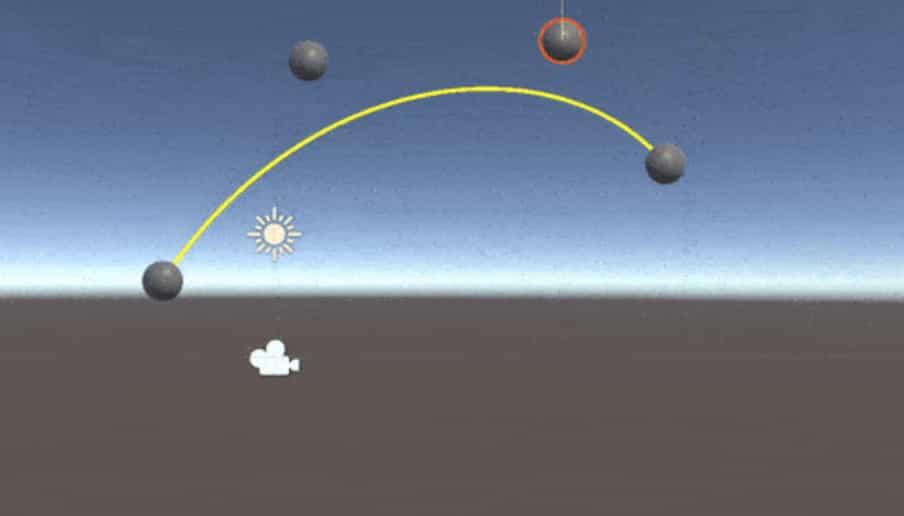
Sometimes, you need to draw lines, circles or curves in your Unity games. In these cases, you can use Unity's LineRenderer grade. In this tutorial, we will see how we tin can draw lines, polygons, circles, moving ridge functions, Bézier Curves. And as well we will see how we can do a free cartoon using Line Renderer in Unity3D. In order to encounter our other Unity tutorials, click here.
Line Renderer Component
To depict a line nosotros have to add a LineRenderer component to a game object. Even if this component can exist fastened to whatsoever game object, I advise you lot create an empty game object and add together the LineRenderer to this object.
Nosotros need a material which volition be assigned to LineRenderer. To do this create a material for the line in Project Tab. Unlit/Color shader is suitable for this material.
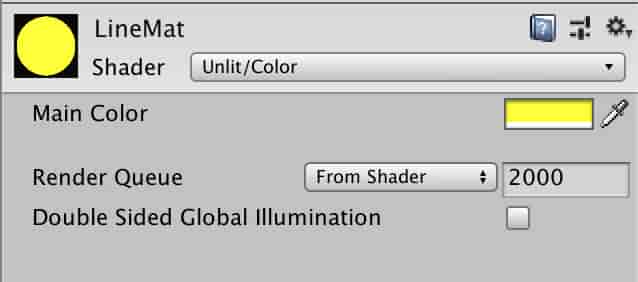
Assign LineMat material to the Line Renderer component.
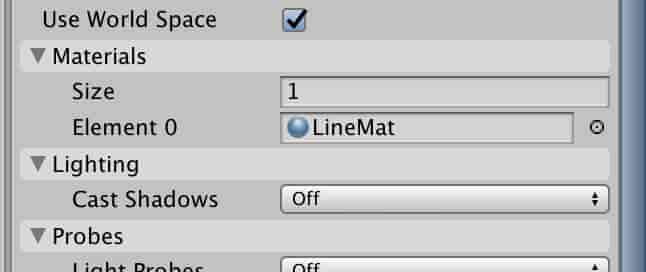
Line Renderer draws lines between determined positions. In other words, nosotros tell the Line Renderer the points which will be connected and Line Renderer connects these points.
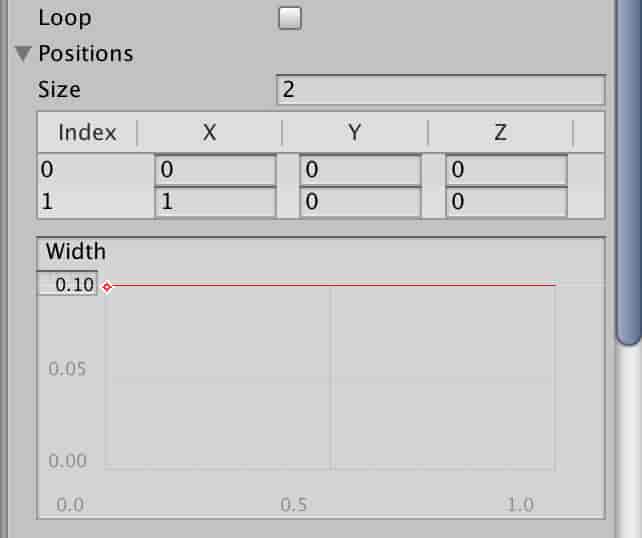
In the Positions section, you can alter the number of points and positions of points. If you enter two different points, you volition go a direct line. You tin also modify the width of the line in the department beneath.
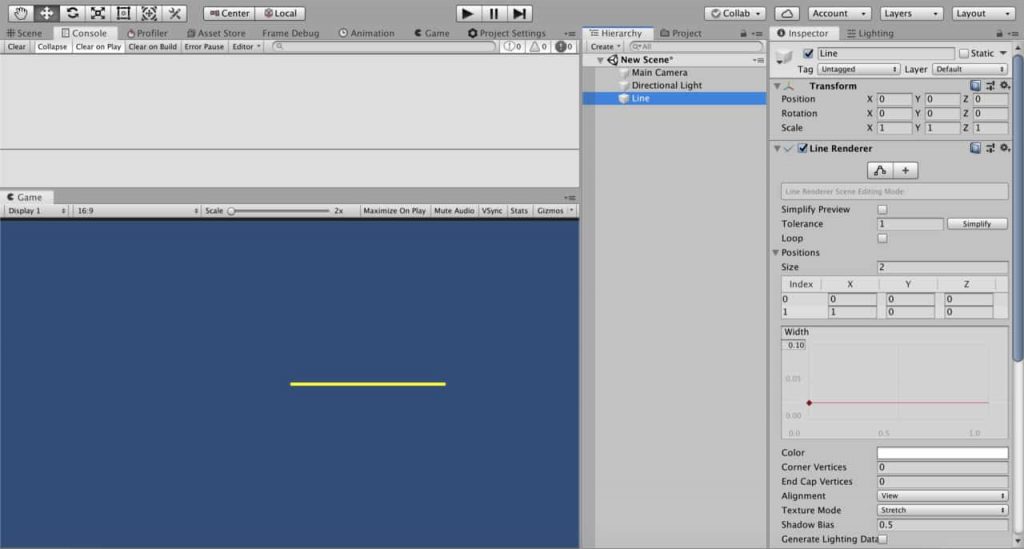
As well, ii draw a triangle, yous need iii points and to describe a rectangle you need 4 points. Let's draw a rectangle as an example.
To draw a rectangle, we need to set positions of 4 points. Nosotros as well have to bank check the Loop toggle to obtain a airtight shape.
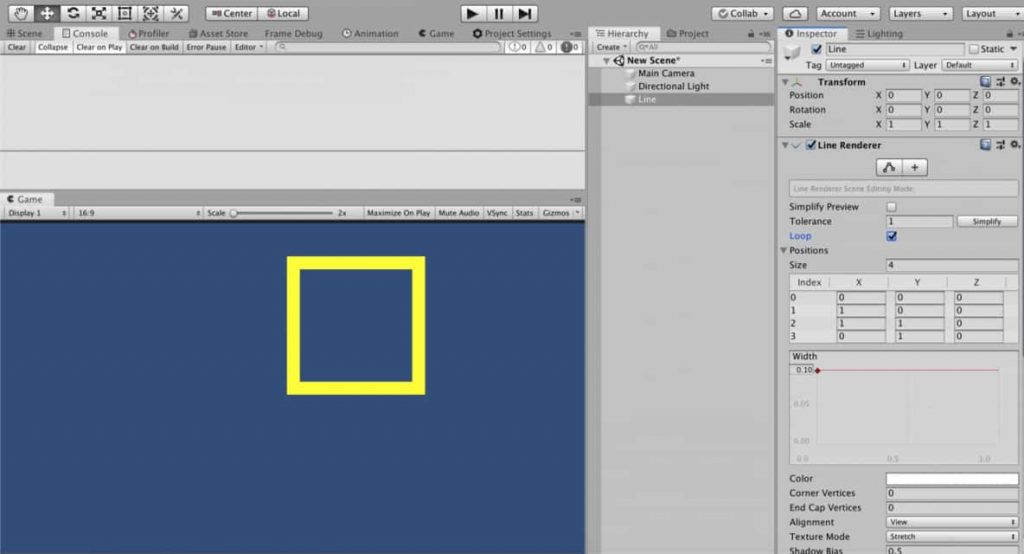
Drawing Lines From C# Script
If nosotros desire to draw or control lines in real-fourth dimension, we demand to create a C# script. To draw lines from a script, we determine the size of position array and coordinates of positions in C# script. Therefore, LineRenderer tin can connect the points.
Permit's draw a triangle using a script equally an example. Showtime, create a script with the name "DrawScript". And attach this script to a game object which already has a LineRenderer component.
public grade DrawScript : MonoBehaviour { private LineRenderer lineRenderer; void Start( ) { lineRenderer = GetComponent<LineRenderer> ( ) ; Vector3[ ] positions = new Vector3[ three ] { new Vector3( 0 , 0 , 0 ) , new Vector3( - 1 , 1 , 0 ) , new Vector3( ane , 1 , 0 ) } ; DrawTriangle(positions) ; } void DrawTriangle(Vector3[ ] vertexPositions) { lineRenderer.positionCount = 3 ; lineRenderer.SetPositions(vertexPositions) ; } }
This script will draw a triangle. Notation that we already ready the line width to 0.1 and checked the loop toggle, earlier. Therefore the aforementioned setting is also valid here.
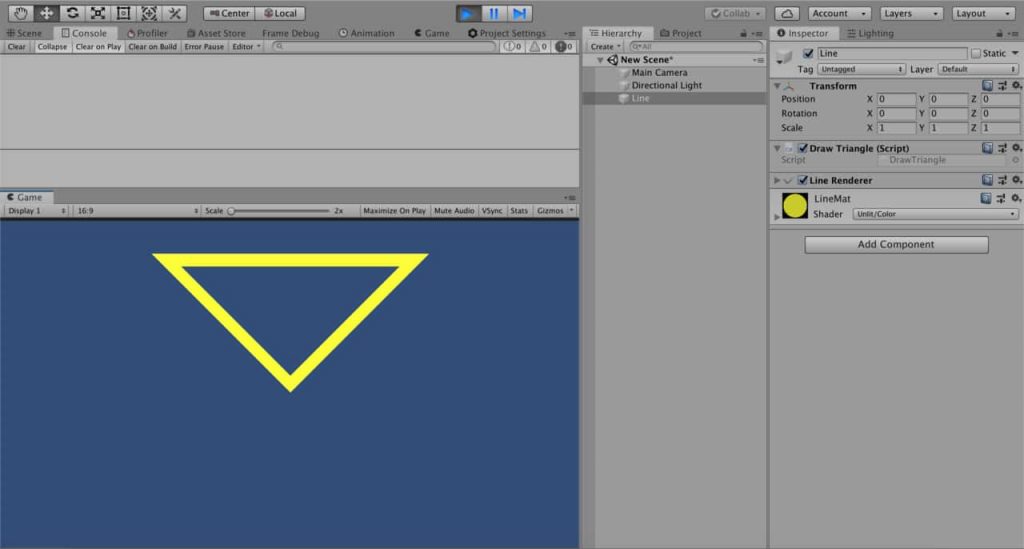
We can likewise alter the line width from the script using startWidth and endWidth. In addition to this, if you would like to change line width past position, you can set unlike values to them. In this case, Line Renderer will interpolate the line width according to position.
public form DrawScript : MonoBehaviour { individual LineRenderer lineRenderer; void Start( ) { lineRenderer = GetComponent<LineRenderer> ( ) ; Vector3[ ] positions = new Vector3[ 3 ] { new Vector3( 0 , 0 , 0 ) , new Vector3( - one , i , 0 ) , new Vector3( 1 , 1 , 0 ) } ; DrawTriangle(positions, 0.02 f , 0.02 f ) ; } void DrawTriangle(Vector3[ ] vertexPositions, float startWidth, bladder endWidth) { lineRenderer.startWidth = startWidth; lineRenderer.endWidth = endWidth; lineRenderer.loop = truthful ; lineRenderer.positionCount = 3 ; lineRenderer.SetPositions(vertexPositions) ; } }
Drawing Regular Polygons and Circles
In this section, we are going to encounter how we can write a method that draws regular polygons. Since circles are n-gons which has big n, our role will be useful for circles likewise. But first, let me explicate the mathematics behind it.
Vertices of regular polygons are on a circle. Also, the center of the circle and the middle of the polygon are top of each other. The nigh reliable method to draw a polygon is to find the angle between successive vertices and locate the vertices on the circumvolve. For instance, angle of the arc between successive vertices of a pentagon is 72 degrees or for an octagon, it is 45 degrees. To notice this bending, nosotros can divide 360 degrees(or 2xPI radians) with the number of vertices.
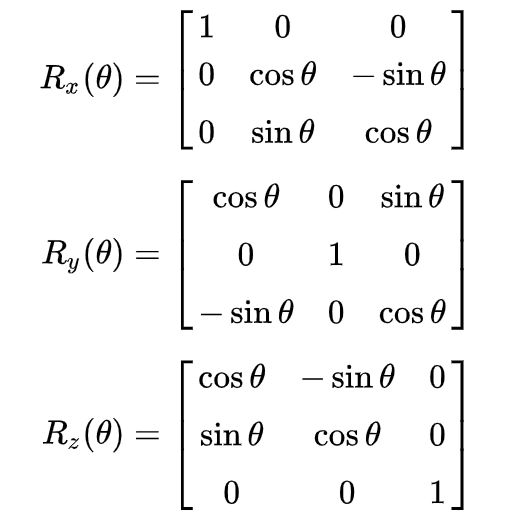
So we need to find the positions of the vertices. To do this nosotros assign an initial point for the outset vertex and rotate this vertex for each vertex using a rotation matrix.
Every bit yous probably know, in social club to rotate a bespeak effectually an centrality, we multiply the position vector of the betoken with the rotation matrix. Rotation matrices for rotations around 10, y and z axes are given on the right.
For case, when nosotros want to rotate a point past 90 degrees around the z-centrality, which has a coordinate (ane,0,0), we multiply the position vector by a rotation matrix.

We need to construct a rotation matrix to rotate each vertex around the z-axis. Allow'south me write our DrawPolygon method first and explain it.
void DrawPolygon( int vertexNumber, float radius, Vector3 centerPos, float startWidth, float endWidth) { lineRenderer.startWidth = startWidth; lineRenderer.endWidth = endWidth; lineRenderer.loop = truthful ; float angle = 2 * Mathf.PI / vertexNumber; lineRenderer.positionCount = vertexNumber; for ( int i = 0 ; i < vertexNumber; i+ + ) { Matrix4x4 rotationMatrix = new Matrix4x4( new Vector4(Mathf.Cos(bending * i) , Mathf.Sin(angle * i) , 0 , 0 ) , new Vector4( - i * Mathf.Sin(angle * i) , Mathf.Cos(angle * i) , 0 , 0 ) , new Vector4( 0 , 0 , 1 , 0 ) , new Vector4( 0 , 0 , 0 , 1 ) ) ; Vector3 initialRelativePosition = new Vector3( 0 , radius, 0 ) ; lineRenderer.SetPosition(i, centerPos + rotationMatrix.MultiplyPoint(initialRelativePosition) ) ; } }
You may wonder why the constructed rotation matrix is iv×4. In calculator graphics, the 3-dimensional world is represented as four-dimensional but this topic is not related to our business here. Nosotros just utilize it as if it is 3-dimensional.
We set the position of initial vertex and rotate it using rotationMatrix each time and add the centre position to information technology.
The following image is an case of a hexagon which is drawn by this method.
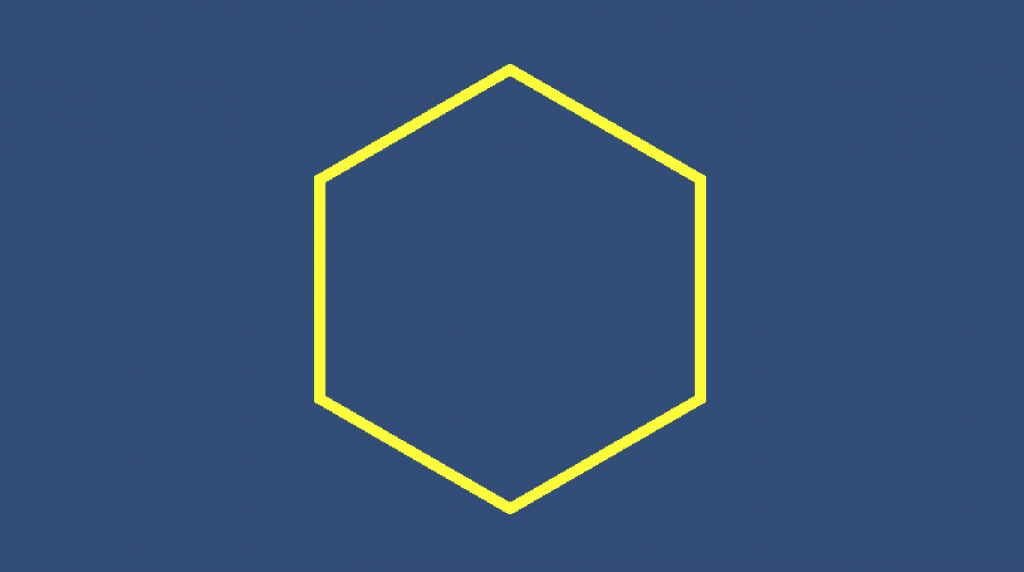
If you increase the number of vertex points, this polygon turns to a circumvolve.
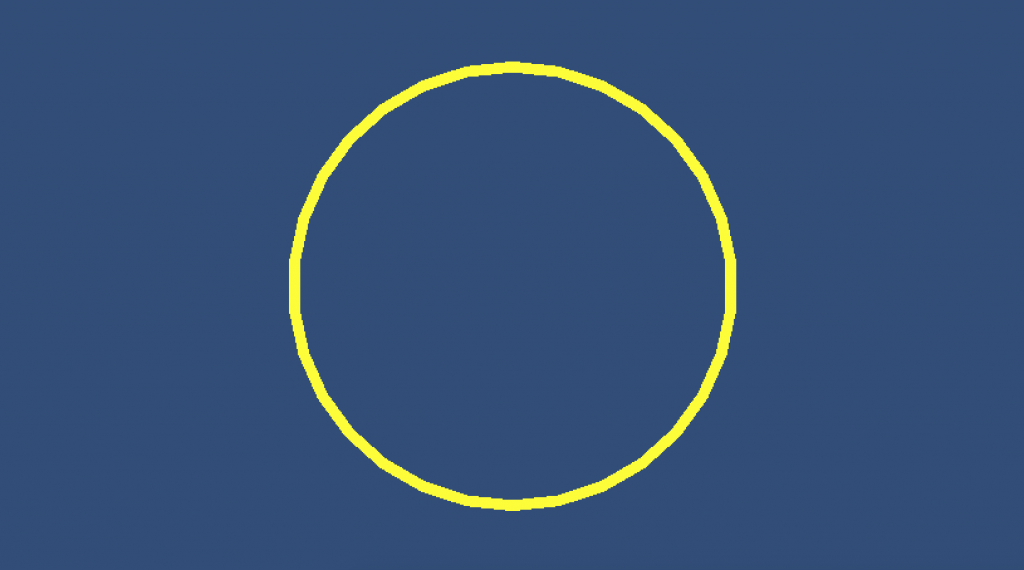
Drawing Waves
In this section, we are going to draw a sinusoidal moving ridge, a traveling moving ridge and a standing wave using sine function.
The mathematical function of the sine moving ridge is given by the post-obit:
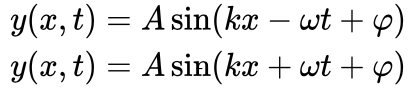
where
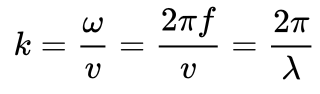
Here, chiliad is wave number, f is frequency, ω is the angular frequency, λ is wavelength, v is the linear speed, t is the time and φ is the stage bending. We will not worry about the phase angle in our discussions.
Sine wave equation with a minus sign represents traveling wave from left to correct and the equation with plus sign represents a traveling line wave correct to left.
In social club to describe a stable sinusoidal wave, we tin drop the fourth dimension part. The following method will draw a sine wave.
void DrawSineWave(Vector3 startPoint, float amplitude, float wavelength) { float 10 = 0f; float y; float k = 2 * Mathf.PI / wavelength; lineRenderer.positionCount = 200 ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { x + = i * 0.001 f ; y = amplitude * Mathf.Sin(k * 10) ; lineRenderer.SetPosition(i, new Vector3(x, y, 0 ) + startPoint) ; } }
Our DrawSineWave method takes three parameters. They are startPoint which is for setting the start position in world space, aamplitude which is for setting the amplitude of the moving ridge and wavelength which is for setting the wavelength of the sine moving ridge.
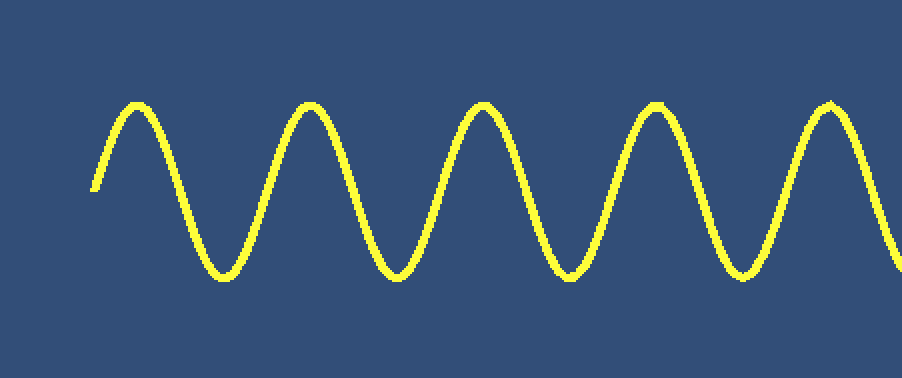
To obtain the positions of the corresponding mathematical function, commencement, we determine the positions on the x-axis. For each 10, we have to calculate the y-position.
To breathing this wave, we accept to implement fourth dimension to our office as follows:
void DrawTravellingSineWave(Vector3 startPoint, float amplitude, float wavelength, bladder waveSpeed) { float 10 = 0f; float y; float k = two * Mathf.PI / wavelength; float w = one thousand * waveSpeed; lineRenderer.positionCount = 200 ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { x + = i * 0.001 f ; y = aamplitude * Mathf.Sin(k * x + w * Time.fourth dimension) ; lineRenderer.SetPosition(i, new Vector3(x, y, 0 ) + startPoint) ; } }
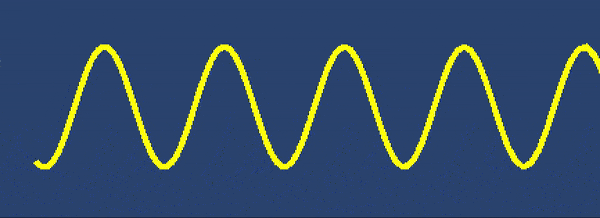
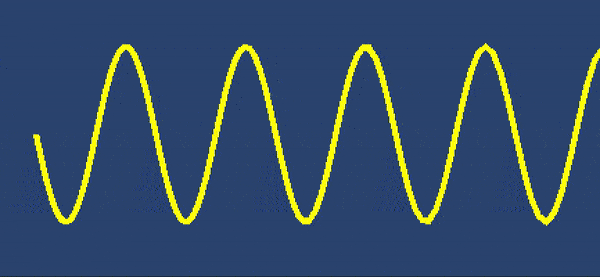
This time we have four parameters. The quaternary parameter is to fix the wave speed. This wave travels to the left since we used plus sign in the function. If nosotros would similar to create a moving ridge that travels to the right, we have to use the minus sign. You should proceed in mind that we have to write this method in Update().
To create a standing moving ridge, we accept to add two waves which travel to the right and which travel to left.
void DrawStandingSineWave(Vector3 startPoint, float amplitude, float wavelength, bladder waveSpeed) { float x = 0f; float y; float k = 2 * Mathf.PI / wavelength; float w = m * waveSpeed; lineRenderer.positionCount = 200 ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { ten + = i * 0.001 f ; y = aamplitude * (Mathf.Sin(one thousand * ten + w * Time.fourth dimension) + Mathf.Sin(m * x - westward * Time.time) ) ; lineRenderer.SetPosition(i, new Vector3(10, y, 0 ) + startPoint) ; } }
Drawing Bézier Curves
Bézier curves are parametric curves that are used to create smooth curved shapes. They are widely used in computer graphics. In this section, nosotros are going to encounter how we can draw Bézier curves.
When a Bézier curve is controlled by 3 points, then it is called Quadratic Bézier Bend(the first equation below) and when information technology is controlled past 4 points, it is called Cubic Bézier Curve.


The following script will draw a quadratic Bézier curve using positions p0, p1, and p2. Y'all should create three game objects and assign these objects to corresponding variables in the script to change the shape of the curve in real-fourth dimension.
using System.Collections; using System.Collections.Generic; using UnityEngine; public class BezierScript : MonoBehaviour { individual LineRenderer lineRenderer; public Transform p0; public Transform p1; public Transform p2; void Start( ) { lineRenderer = GetComponent<LineRenderer> ( ) ; } void Update( ) { DrawQuadraticBezierCurve(p0.position, p1.position, p2.position) ; } void DrawQuadraticBezierCurve(Vector3 point0, Vector3 point1, Vector3 point2) { lineRenderer.positionCount = 200 ; bladder t = 0f; Vector3 B = new Vector3( 0 , 0 , 0 ) ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { B = ( i - t) * ( 1 - t) * point0 + 2 * ( i - t) * t * point1 + t * t * point2; lineRenderer.SetPosition(i, B) ; t + = ( 1 / ( float )lineRenderer.positionCount) ; } } }
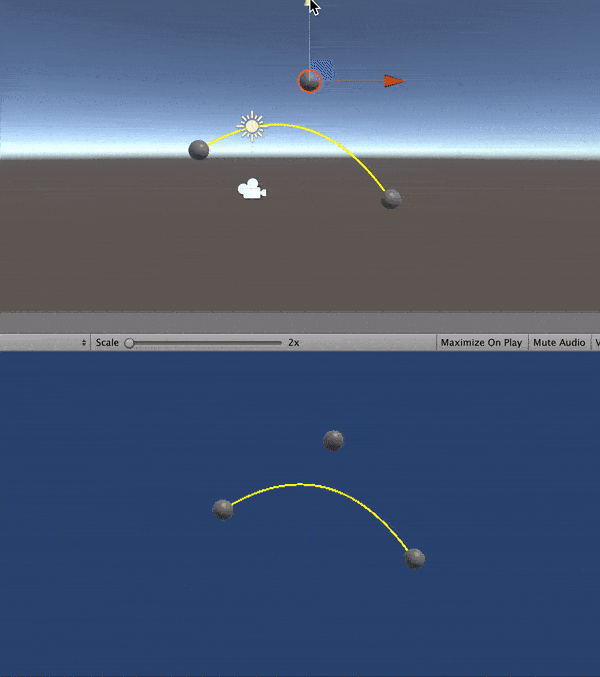
Also, the following method draws a cubic Bézier curve. This fourth dimension nosotros need 4 points.
void DrawCubicBezierCurve(Vector3 point0, Vector3 point1, Vector3 point2, Vector3 point3) { lineRenderer.positionCount = 200 ; float t = 0f; Vector3 B = new Vector3( 0 , 0 , 0 ) ; for ( int i = 0 ; i < lineRenderer.positionCount; i+ + ) { B = ( ane - t) * ( 1 - t) * ( 1 - t) * point0 + three * ( ane - t) * ( 1 - t) * t * point1 + 3 * ( 1 - t) * t * t * point2 + t * t * t * point3; lineRenderer.SetPosition(i, B) ; t + = ( ane / ( float )lineRenderer.positionCount) ; } }
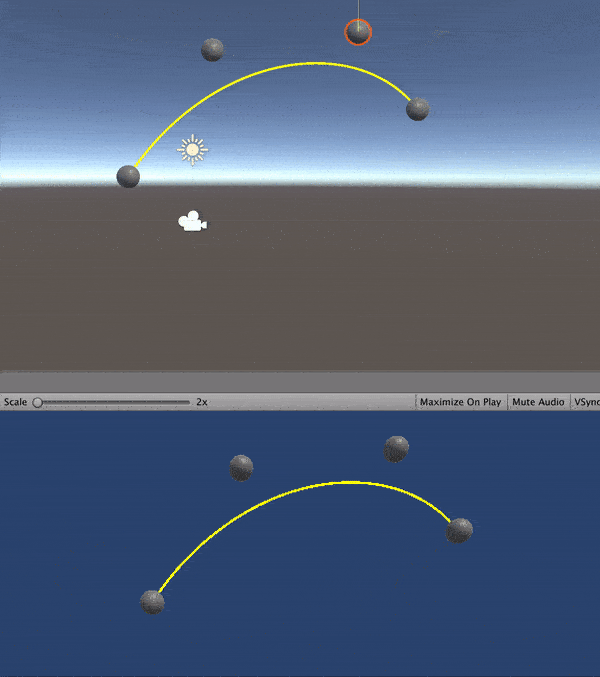
Gratis Drawing using Line Renderer
In this section, we are going to come across how we can describe freely using the mouse position. We can do this past creating a new game object with a line renderer attached. When we press the left mouse button, a new game object is created and each frame the position of the mouse added to the line renderer.

First of all, we need a prefab to create a new game object when nosotros press the left mouse push button. This is an empty game object with a line renderer component attached. In addition to this, do not forget to assign a material to the line renderer component. Create a prefab from this game object.
Second, create an empty game object and adhere the post-obit script DrawManager.
using System.Collections; using System.Collections.Generic; using UnityEngine; public grade DrawManager: MonoBehaviour { individual LineRenderer lineRenderer; public GameObject drawingPrefab; void Update( ) { if (Input.GetMouseButtonDown( 0 ) ) { GameObject drawing = Instantiate(drawingPrefab) ; lineRenderer = cartoon.GetComponent<LineRenderer> ( ) ; } if (Input.GetMouseButton( 0 ) ) { FreeDraw( ) ; } } void FreeDraw( ) { lineRenderer.startWidth = 0.1 f ; lineRenderer.endWidth = 0.i f ; Vector3 mousePos = new Vector3(Input.mousePosition.x, Input.mousePosition.y, 10f) ; lineRenderer.positionCount+ + ; lineRenderer.SetPosition(lineRenderer.positionCount - ane , Camera.main.ScreenToWorldPoint(mousePos) ) ; } }
When yous press the left mouse button, a new game object is instantiated from the prefab which we created before. We become the line renderer component from this game object. Then while we are pressing the left mouse push, we call FreeDraw() method.
In the FreeDraw method, we take x and y components of the mouse position and ready the z-position every bit 10. Here, the mouse position is in the screen infinite coordinates. But we employ world space coordinates in line renderer. Therefore we demand to catechumen mouse position to world infinite coordinates. In each frame, we also need to increase the number of points. Since nosotros do non know how many points nosotros need, we cannot fix position count before.
References
one-https://docs.unity3d.com/ScriptReference/LineRenderer.html
2-http://www.theappguruz.com/blog/bezier-bend-in-games
3-https://en.wikipedia.org/wiki/Bézier_curve
4-https://en.wikipedia.org/wiki/Sine_wave
Source: https://www.codinblack.com/how-to-draw-lines-circles-or-anything-else-using-linerenderer/
0 Response to "dotted line drawings 3d for mom"
Post a Comment